Grid Layout in Android is best for displaying your data in grid. You may argue me that you can use nested Linear Layout to display your data in grid. But the problem is that nested Linear Layout is hard to handle when you want to merge cell in grid. the more merged cell you have, the more complex you have to work using nest linear layout.
However, when you decided to use grid layout, the grid column and row size may not be distributed equally. Sometimes the size will automatically reduces to zero if there is no data on entire row or column.
In this tutorial, I will guide you on how to distribute the grid layout row and column size equally like this
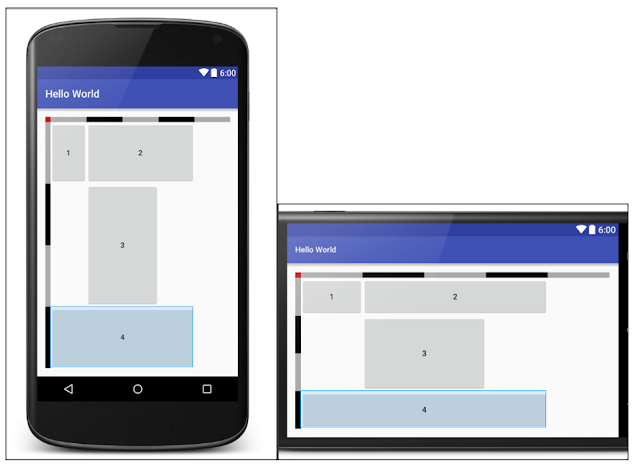
1In file
2To make the grid layout compatible with old android version, you should add this code in your build.gradle file of application module
3Now, it's time to add grid layout. Open your layout XML File and place this code
4This step is to add row/column guide. This is used for debugging only.
Place this code inside GridLayout
dimens.xml
of your project, set gridLayout_debugColumnSize
value to be size of the column guide. This is for debugging only. You can set this value to zero after finish debugging.compile 'com.android.support:gridlayout-v7:(yourLastestSDKVersion)'
<android.support.v7.widget.GridLayout android:layout_width="match_parent" android:layout_height="match_parent" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentTop="true" app:columnCount="(numberOfColumnsYouWant + 1)" app:rowCount="(numberOfRowsYouWant + 1)"> </android.support.v7.widget.GridLayout>
Place this code inside GridLayout
<TextView android:background="@android:color/darker_gray" app:layout_gravity="fill_horizontal" app:layout_columnWeight="1" app:layout_rowWeight="0" app:layout_column="(running column number from 1 to max column number)" app:layout_row="0" android:height="@dimen/gridLayout_debugColumnSize" android:text=""/>
<TextView android:background="@android:color/darker_gray" app:layout_gravity="fill_vertical" app:layout_columnWeight="0" app:layout_rowWeight="1" app:layout_column="0" app:layout_row="(running row number from 1 to max row number)" android:width="@dimen/gridLayout_debugColumnSize" android:text=""/>
cell[0][0]
, use this code instead<TextView android:background="@android:color/holo_red_dark" app:layout_columnWeight="0" app:layout_rowWeight="0" app:layout_column="0" app:layout_row="0" android:width="@dimen/gridLayout_debugColumnSize" android:height="@dimen/gridLayout_debugColumnSize" android:text=""/>
<yourControlName app:layout_column="(coloumn position you want)" app:layout_row="(row position you want)" app:layout_columnSpan="(column span size)" app:layout_rowSpan="(row span size)" app:layout_gravity="fill" android:layout_width="0dp" android:layout_height="0dp" />
gridLayout_debugColumnSize
value in step 1 to 0dp
.Full code
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.piyapan039285.helloworld.GridLayoutMainActivity"> <android.support.v7.widget.GridLayout android:layout_width="match_parent" android:layout_height="match_parent" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentTop="true" app:columnCount="6" app:rowCount="5" android:id="@+id/android.support.v7.widget.GridLayout"> <TextView android:background="@android:color/holo_red_dark" app:layout_columnWeight="0" app:layout_rowWeight="0" app:layout_column="0" app:layout_row="0" android:width="@dimen/gridLayout_debugColumnSize" android:height="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/darker_gray" app:layout_gravity="fill_horizontal" app:layout_columnWeight="1" app:layout_rowWeight="0" app:layout_column="1" app:layout_row="0" android:height="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/black" app:layout_gravity="fill_horizontal" app:layout_columnWeight="1" app:layout_rowWeight="0" app:layout_column="2" app:layout_row="0" android:height="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/darker_gray" app:layout_gravity="fill_horizontal" app:layout_columnWeight="1" app:layout_rowWeight="0" app:layout_column="3" app:layout_row="0" android:height="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/black" app:layout_gravity="fill_horizontal" app:layout_columnWeight="1" app:layout_rowWeight="0" app:layout_column="4" app:layout_row="0" android:height="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/darker_gray" app:layout_gravity="fill_horizontal" app:layout_columnWeight="1" app:layout_rowWeight="0" app:layout_column="5" app:layout_row="0" android:height="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/darker_gray" app:layout_gravity="fill_vertical" app:layout_columnWeight="0" app:layout_rowWeight="1" app:layout_column="0" app:layout_row="1" android:width="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/black" app:layout_gravity="fill_vertical" app:layout_columnWeight="0" app:layout_rowWeight="1" app:layout_column="0" app:layout_row="2" android:width="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/darker_gray" app:layout_gravity="fill_vertical" app:layout_columnWeight="0" app:layout_rowWeight="1" app:layout_column="0" app:layout_row="3" android:width="@dimen/gridLayout_debugColumnSize" android:text=""/> <TextView android:background="@android:color/black" app:layout_gravity="fill_vertical" app:layout_columnWeight="0" app:layout_rowWeight="1" app:layout_column="0" app:layout_row="4" android:width="@dimen/gridLayout_debugColumnSize" android:text=""/> <Button app:layout_column="1" app:layout_row="1" app:layout_columnSpan="1" app:layout_rowSpan="1" app:layout_gravity="fill" android:layout_width="0dp" android:layout_height="0dp" android:text="1"/> <Button app:layout_column="2" app:layout_row="1" app:layout_columnSpan="3" app:layout_rowSpan="1" app:layout_gravity="fill" android:layout_width="0dp" android:layout_height="0dp" android:text="2"/> <Button app:layout_column="2" app:layout_row="2" app:layout_columnSpan="2" app:layout_rowSpan="2" app:layout_gravity="fill" android:layout_width="0dp" android:layout_height="0dp" android:text="3"/> <Button app:layout_column="1" app:layout_row="4" app:layout_columnSpan="4" app:layout_rowSpan="1" app:layout_gravity="fill" android:layout_width="0dp" android:layout_height="0dp" android:text="4"/> </android.support.v7.widget.GridLayout> </RelativeLayout>
No comments:
Post a Comment